How to Train an AI Assistant in JavaScript (With OpenAI + Your Own Data)
Train your own AI assistant in JavaScript using OpenAI’s API. This guide walks through setup, code, and connecting it to real workflows.
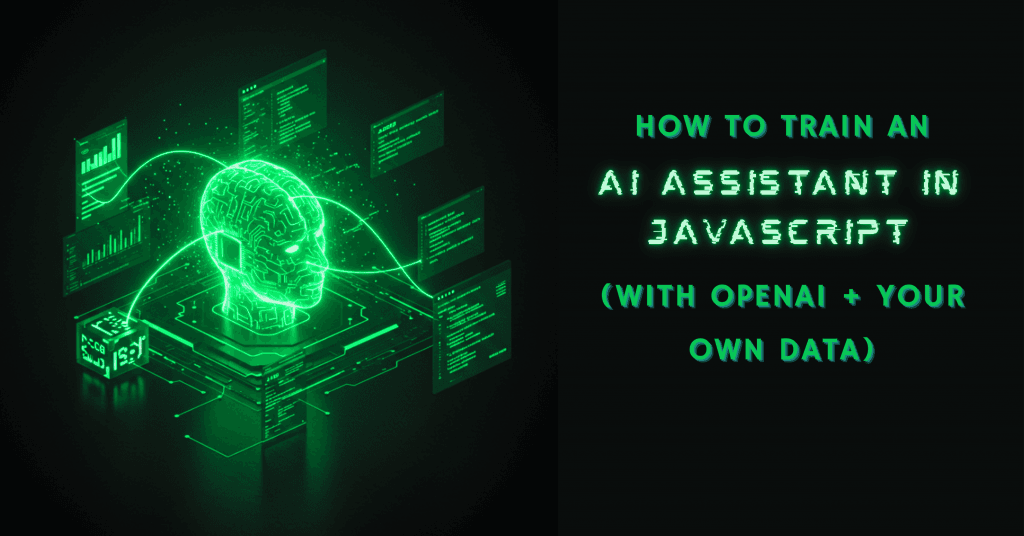
Introduction
If you’ve ever wanted to build your own AI assistant but didn’t know where to start, this guide is for you. We’ll walk through how to train an AI assistant in JavaScript using simple tools. No machine learning background is needed.
You won’t be building a model from scratch. Instead, you’ll use OpenAI’s API as the assistant’s brain. Then you’ll write a Node.js app that sends messages to the model and gets smart replies back. You’ll also learn how to give your assistant its own knowledge so it can answer questions based on your data.
This is a hands-on tutorial. You’ll write real code, test real responses, and build something functional. Whether you want a support bot, a personal tool, or a prototype for your startup, you’ll have a working AI assistant by the end.
What You’ll Build
- A Node.js server that connects to OpenAI’s GPT model
- A custom AI assistant that responds with your content
- A simple setup that you can expand or plug into other apps
You’ll define your assistant’s role, give it data to work with, and handle conversations—all in JavaScript.
Who This Is For
This guide is for developers, indie hackers, and anyone technical enough to write JavaScript. You don’t need to know anything about machine learning. You just need to know how to write and run basic code.
Let’s jump in and learn how to train an AI assistant in JavaScript step by step.
What Does It Actually Mean?
When people search for how to train an AI assistant in JavaScript, they often imagine complex machine learning models, neural networks, or huge datasets. That’s not what we’re doing here.
In this guide, “training” means giving your assistant clear instructions, custom information, and behavior logic using code. We’re using the OpenAI API, which already handles the hard part—the language understanding and generation. Your job is to guide the assistant’s responses using prompts and data.
This type of training is fast, flexible, and powerful. You don’t need to fine-tune a model. You just shape the way your assistant responds by feeding it content and writing a simple prompt structure.
Types of “Training” You’ll Do in JavaScript
Here are the three simple ways we’ll train your AI assistant using JavaScript:
- System prompt training
You tell the assistant what kind of role it should play, like “You are a helpful support assistant.” - Data injection
You load structured data like FAQs, docs, or internal notes into the prompt. The assistant then uses that info to answer. - Context control
You build logic in JavaScript that manages the conversation flow—like remembering the last question, or summarizing a long message.
What You Need to Start Training an AI Assistant in JavaScript
If you’re learning how to train an AI assistant in JavaScript, the good news is that your tool stack can be simple and effective. You won’t need machine learning frameworks or complex infrastructure. Just a clean JavaScript backend, an API key, and some custom data.
Here are the five tools you’ll need, and how each one fits into the process of building your assistant.
Node.js + Express for the Backend
Node.js lets you write JavaScript on the server side. Express is a fast way to build a lightweight API for your assistant. You’ll use these to receive messages from users, format prompts, and send those prompts to OpenAI.
Here’s a basic example of a working Express setup:

OpenAI API for Language Processing
This is the assistant’s brain. OpenAI‘s chat-completion
endpoint lets you send a series of messages and receive intelligent replies. You don’t need to train a model. You simply structure the conversation using a few roles:

You’ll send these messages in your API call. The GPT model will generate the assistant’s reply based on this history.
This is the core of how to train an AI assistant in JavaScript—not by editing the model, but by feeding it the right input.
JSON or Text Files for Custom Data
To make the assistant useful, it needs real information. You’ll simulate training by loading structured content from a JSON file.

In your JavaScript code, you’ll read this file and inject it into the prompt sent to GPT. This gives your assistant real knowledge without needing a database or vector index.
Optional Frontend to Test the Assistant
If you want a simple UI, you can build one in HTML or React. It doesn’t affect how you train the AI, but it helps with testing.
Example (HTML + fetch):

You can plug this into your local project for fast testing as you train your AI assistant.
Dotenv to Handle Your API Key
You’ll get an API key from OpenAI, but you should never hardcode it. Use dotenv
to manage it safely:

Then create a .env
file:

In your code:

With these five tools, you’ll be fully set up to follow the next steps in how to train an AI assistant in JavaScript—and turn a basic idea into a working assistant.
Step-by-Step: How to Train an AI Assistant in JavaScript (with Working Code)
How to Train an AI Assistant in JavaScript Using OpenAI and Custom Data
Now let’s put everything together and build a working AI assistant. In this section, you’ll learn how to train an AI assistant in JavaScript by:
- Setting up your Node.js environment
- Connecting to the OpenAI API
- Feeding your assistant with your own content
- Generating smart, contextual responses
You’ll end up with a real assistant you can test, use, or integrate into a bigger app.
Step 1 – Set Up the Server (Express + dotenv)
Create your project folder and install the necessary packages:

Set up your .env
file for your API key:

Now create your server.js
:

Step 2 – Load and Format Your “Training Data”
Simulate training by loading custom content from a local JSON file (e.g. faq.json
):

In your server.js
, read and format it:

Step 3 – Build the Prompt and Send to OpenAI
Here’s the heart of how to train an AI assistant in JavaScript: prompt engineering. Combine the assistant’s role, your data, and the user’s message into one prompt.

Step 4 – Test Your AI Assistant
Run your server:

Now you can test your assistant with a tool like Postman, Insomnia, or a simple frontend. Example payload:

The assistant will search the loaded FAQ data and respond based on it—no training, no database, just smart prompt injection.
That’s How You Train an AI Assistant in JavaScript
You’ve now seen exactly how to train an AI assistant in JavaScript using OpenAI’s GPT API and your own structured content. The assistant can pull answers from your FAQ file and act like a real, trained bot.
In the next section, we’ll cover 3 example assistants you can build using this exact framework.
Real-World Use Cases for Your AI Assistant in JavaScript
Once you’ve learned how to train an AI assistant in JavaScript, the next step is using it for something practical. The beauty of this approach is that you can reuse the same framework—Node.js + OpenAI + structured data—for tons of different tasks.
Here are three smart use cases that are easy to build and actually useful.
1. A Customer Support Bot with Custom FAQs
This is the most common use case, and it’s perfect for startups or internal tools.
How it works:
- You load a JSON or Markdown file with FAQs
- The assistant uses that data to answer incoming questions
- You can route this via your site chat, Slack, or email form
Why it works:
- It saves support time
- It stays accurate because you control the content
- You don’t need to retrain it when policies change—just update the file
2. An Internal Help Desk for Your Team
Train your assistant with:
- Onboarding info
- Company policies
- Technical documentation
Let team members ask things like:
“How do I request time off?”
“Where’s the latest product roadmap?”
“What’s the Wi-Fi password?”
This turns your assistant into a private, internal knowledge base—without needing to set up something like Confluence or Notion AI.
3. A Lead Qualification Bot for Forms or Chat
You can also train your assistant to ask follow-up questions when someone submits a form or starts a chat. Example flow:
- User says: “I’m interested in your product”
- Assistant asks: “What’s your use case?”
- Then it tags the lead or passes to a human
Train it with product info, pricing tiers, and qualification criteria, and it becomes a smart pre-sales layer—without needing a CRM integration right away.
Common Pitfalls When You Train an AI Assistant in JavaScript
Learning how to train an AI assistant in JavaScript is simple on paper—but there are a few things that can quietly break your assistant or make it useless. Here are the most common mistakes, and how to avoid them.
1. Overloading the Prompt with Too Much Data
One of the easiest ways to confuse your assistant is by cramming a giant wall of text into the prompt.
The OpenAI API has token limits (around 4,096 tokens for GPT-3.5, and higher for GPT-4), but even below that, long, unstructured context leads to vague or broken replies.
Fix: Be selective with your training data. Use bullet points, concise answers, and format it cleanly. Only include what the assistant really needs to know.
2. Not Giving a Clear System Prompt
If you skip the system
message or keep it too vague, your assistant won’t act the way you expect.
Saying “you are a helpful bot” is not enough. You need to tell it exactly what role it plays and what content to rely on.
Fix: Write specific system prompts like:
“You are a customer support assistant trained on the following company policies and FAQs. Only use that data to answer.”
That’s how you guide the assistant’s tone, limits, and purpose.
3. Assuming It Has Memory
Your assistant doesn’t “remember” past chats unless you manually feed them into the messages array. Every call to the OpenAI API is stateless unless you design a memory loop.
Fix: If you need memory, store the full conversation history (or a summary) and pass it back in as previous messages.
4. No Input Validation or Error Handling
A real-world assistant needs to handle weird input, blank questions, and occasional API issues. Many devs forget this.
Fix: Add basic validation and try/catch logic around your API calls. Failing gracefully is part of a “trained” assistant too.
Avoiding these mistakes will make your build smoother, and your assistant way more reliable. You now know more than most tutorials on how to train an AI assistant in JavaScrip
Final Thoughts: What You Built and What’s Next
By now, you’ve learned exactly how to train an AI assistant in JavaScript using OpenAI, simple code, and your own content. You didn’t need a massive ML stack or complicated infrastructure—just smart prompt design, clear structure, and practical logic.
You now have:
- A working AI assistant powered by OpenAI
- The ability to inject your own data as training context
- A lightweight Node.js backend that you can reuse or scale
This setup works for support bots, internal tools, lead qualifiers, onboarding flows. Basically anything where language and logic meet.
The best part? You’re not locked into one format. You can plug this assistant into:
- A frontend chat widget
- A Slack bot
- An API used by your internal tools
Or you can keep going and upgrade it with:
- Long-term memory
- Voice input/output
- Data lookup from a database or vector store
If you want to go deeper, Part 2 will cover how to expand this into a more dynamic AI product, with persistent memory, smarter context handling, and integration into real workflows.
For now, you’ve done the hard part: you’ve built something real.
Related Articles
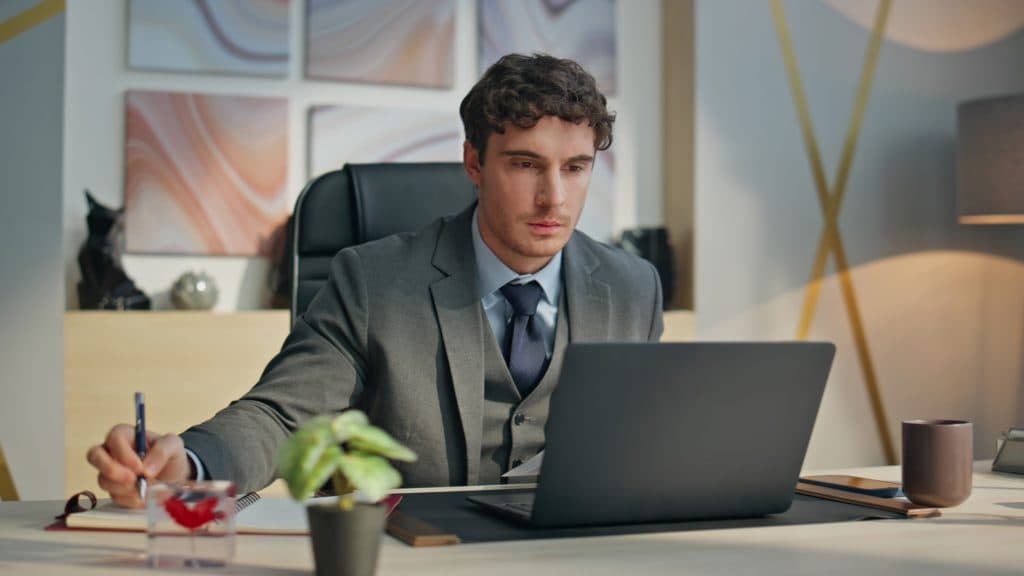
Jun 30, 2025
Read more
Digital Transformation for Business: What You Need to Know
Mastering digital transformation for your business. Get clear steps for tech adoption, growth, and a secure future.
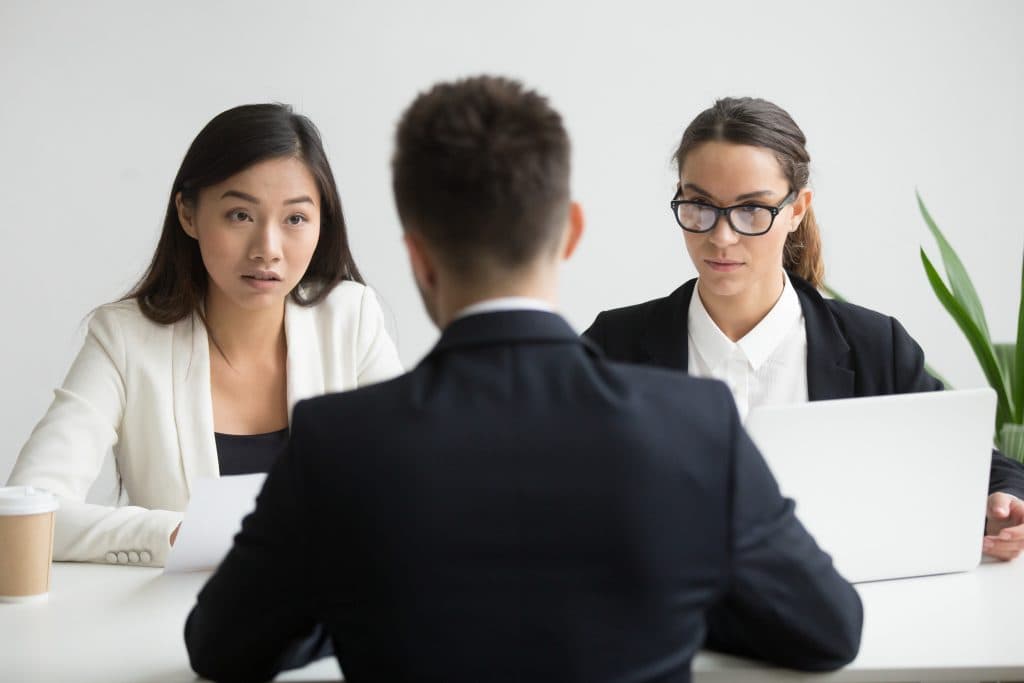
Jun 25, 2025
Read more
The Ultimate Guide to Hiring Remote Developers for Your Tech Team.
Learn to hire remote developers successfully. Discover benefits, overcome challenges, and find top global tech talent with 8Seneca's guide.
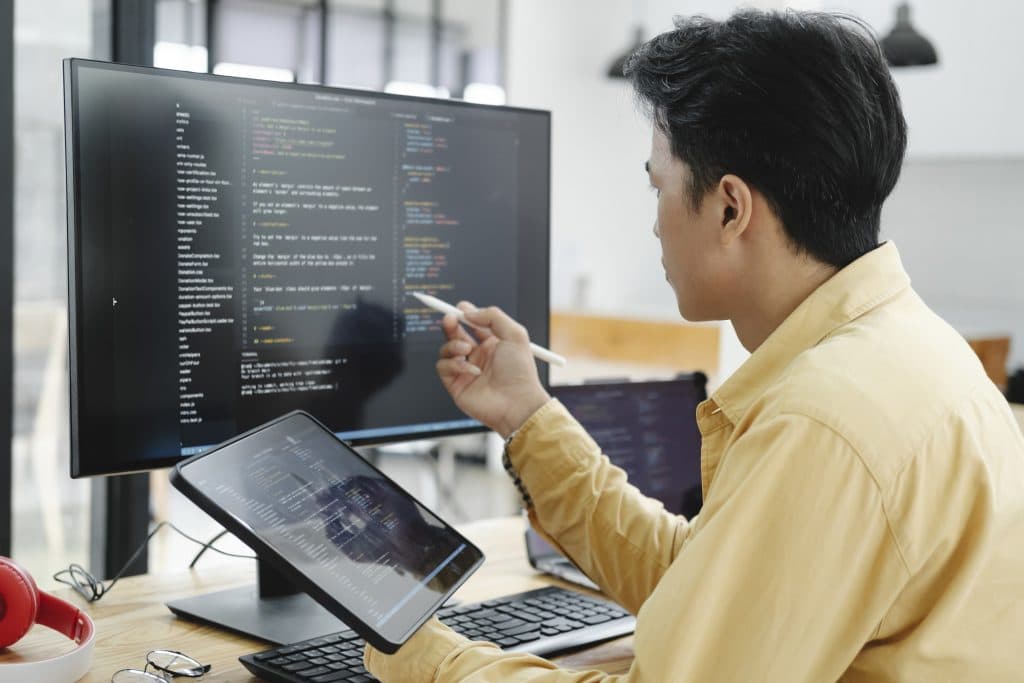
Jun 17, 2025
Read more
Why Vietnamese Full-Stack JavaScript Developers Are Your Next Strategic Hire
Hire top Vietnamese Full-Stack JavaScript developers. 8Seneca connects you to elite talent in Vietnam. Accelerate your project!
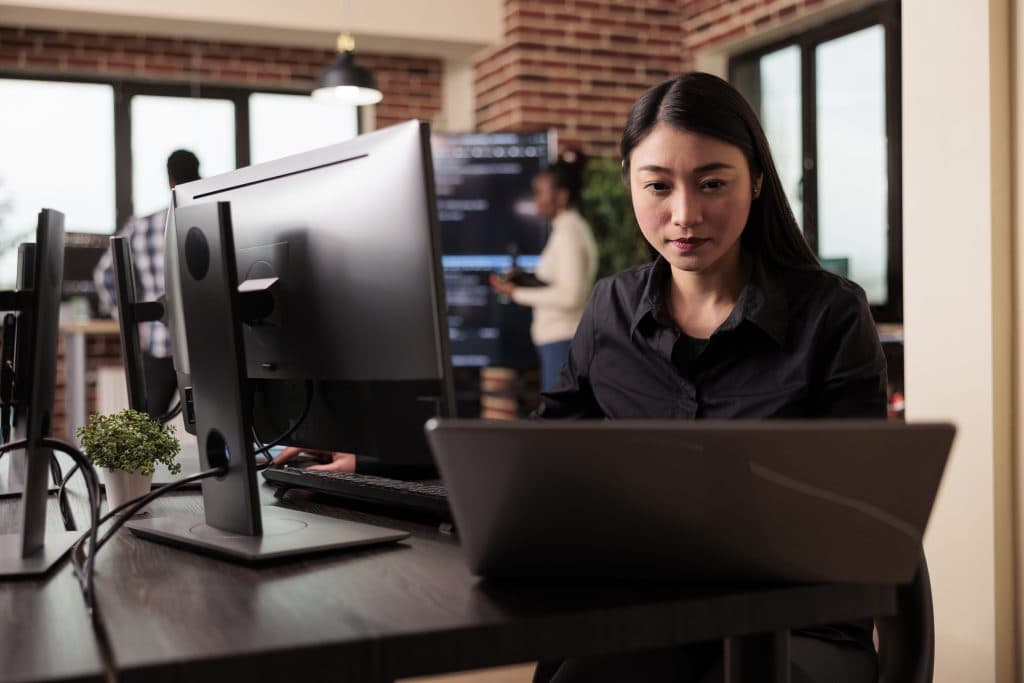
Jun 10, 2025
Read more
What European Clients Often Get Wrong About IT Outsourcing Companies in Vietnam
Many clients misunderstand how it outsourcing companies in Vietnam work. Here’s what to know before hiring in 2025.
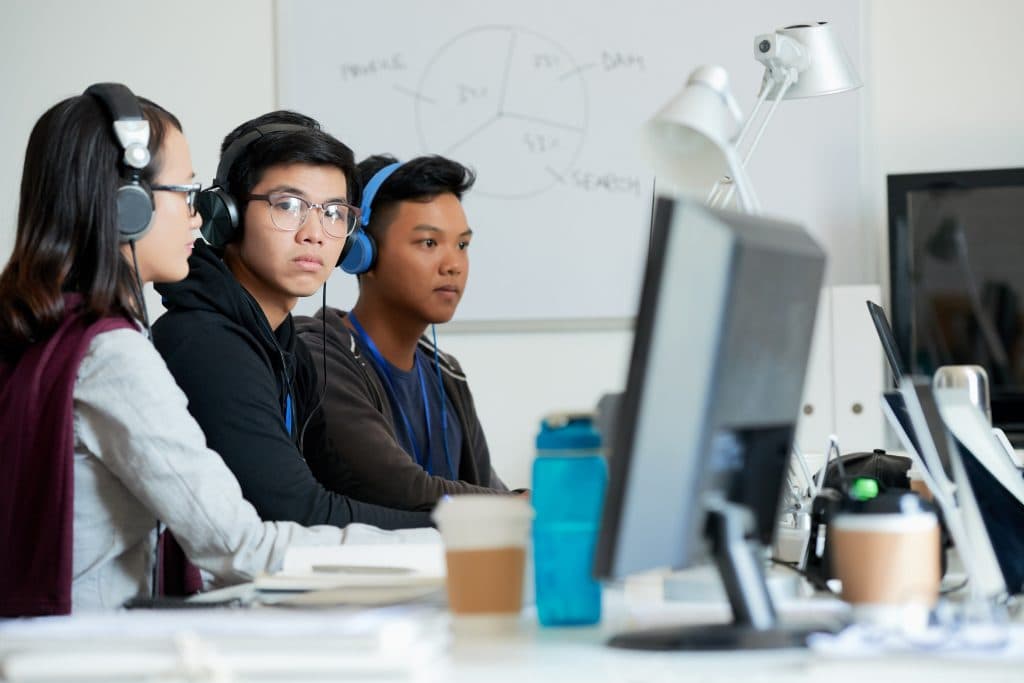
Jun 02, 2025
Read more
Vietnam Software Outsourcing: Why Global Companies Are Turning East in 2025
Vietnam is becoming a top destination for software outsourcing. Learn why global companies are choosing Vietnam for tech projects in 2025.
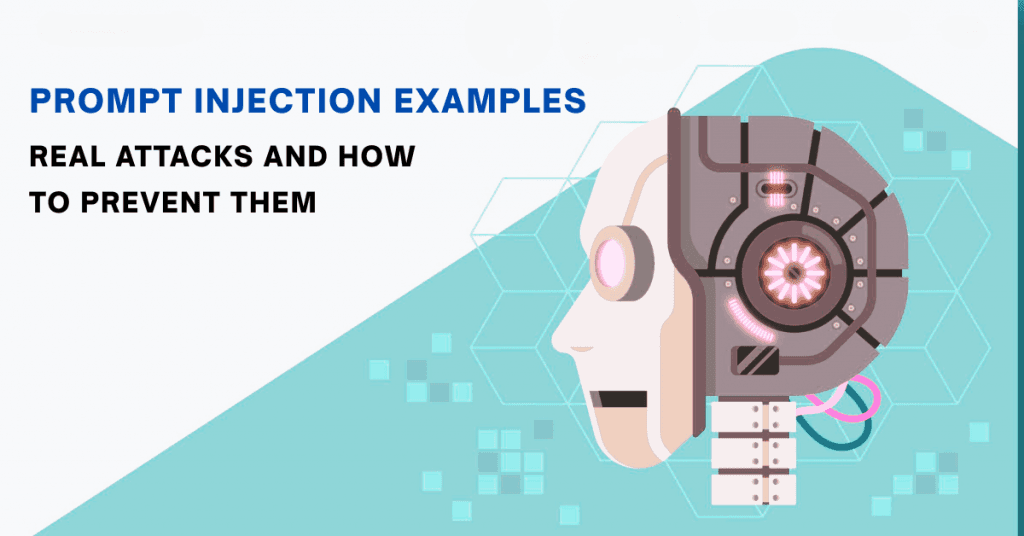
May 05, 2025
Read more
Prompt Injection Examples: Real Attacks and How to Prevent Them
Clear prompt injection examples and attacks explained. Learn what prompt injection is and how to protect your AI systems from these risks.