C# Web Development: Exploring Advanced Concepts
Explore advanced concepts in C# web development, from asynchronous programming to LINQ, enhancing your skills and application efficiency.
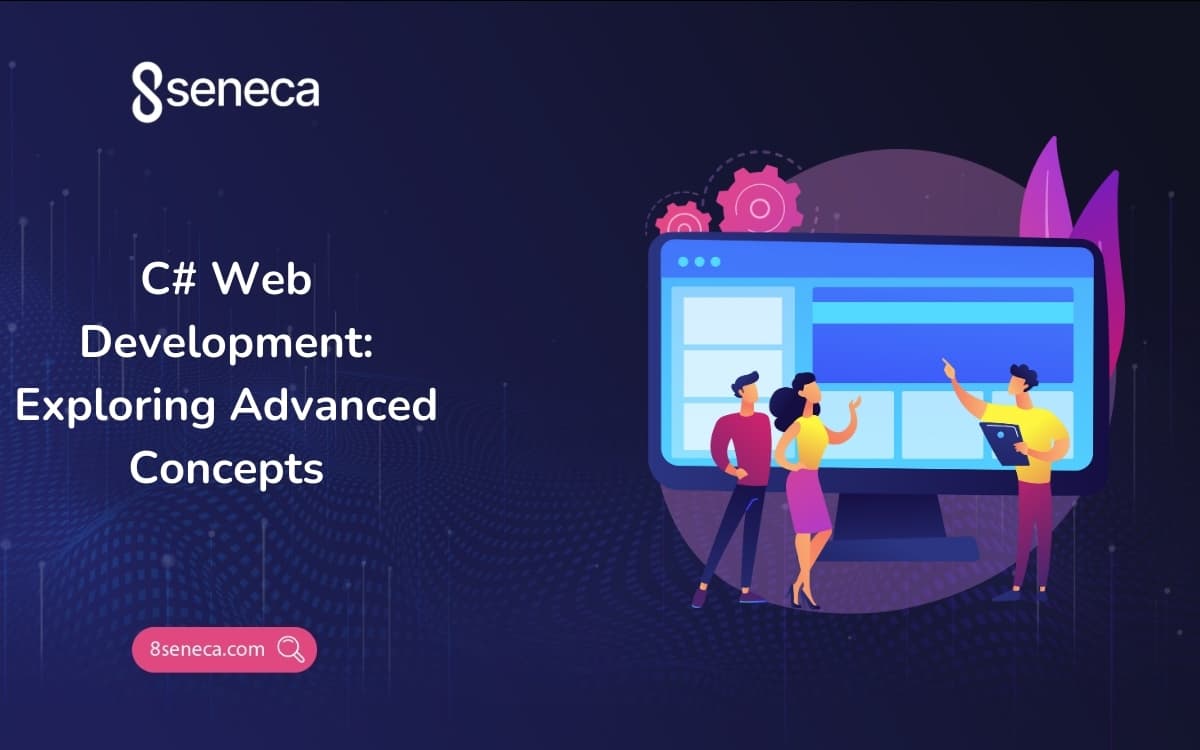
As developers progress in their journey of C# web development, they inevitably encounter advanced concepts. These concepts expand their skill set and enable them to build more sophisticated and efficient web applications. In this comprehensive guide, we’ll delve into an array of advanced concepts in C# web development. We’ll explore their significance, implementation, and real-world applications.
Advanced Concepts in C# Web Development
Generics
Generics in C# offer a powerful mechanism for creating reusable components that work with any data type. By defining classes, interfaces, and methods with type parameters, developers can write generic algorithms and data structures that adapt to different data types at compile time. Widely used in collections, LINQ queries, and custom data structures, generics enhance code flexibility and type safety while maintaining performance and readability.
Delegates and Events
Events and delegates provide robust mechanisms for implementing callbacks and event-driven programming in C#. Delegates act as function pointers, encapsulating method references and enabling dynamic invocation. Events, built upon delegates, allow classes to define and raise events that notify subscribers of specific actions. Essential for implementing observer patterns, asynchronous programming and event-driven architectures, delegates and events facilitate flexible and decoupled code organization.
Lambda Expressions
Lambda expressions introduce a concise syntax for defining anonymous methods and inline functions in C#. By eliminating the need for separate method definitions, lambda expressions enhance code readability and reduce boilerplate. Commonly employed in LINQ queries, event handlers and asynchronous programming, lambda expressions enable developers to write expressive and functional-style code with ease.
Asynchronous Programming
Traditional synchronous programming models can often lead to blocking operations, hindering application responsiveness and scalability. However, asynchronous programming techniques in C# such as async/await, allow developers to execute non-blocking I/O operations efficiently. By leveraging asynchronous programming, developers can enhance application performance. They can improve user experience and optimize resource utilization. Particularly, this is beneficial in scenarios involving web requests, file I/O and database access.
LINQ (Language Integrated Query)
LINQ, a potent feature in C#, empowers developers to query data from diverse sources through a unified syntax. Moreover, with LINQ, developers can execute intricate queries on collections, arrays databases and XML data. Its seamless integration of querying capabilities into C# code facilitates streamlined development processes. By leveraging LINQ, developers can create succinct, expressive and type-safe queries. As a result, they can enhance code readability, maintainability and productivity within C# web applications.
Entity Framework Core
Entity Framework Core is an object-relational mapping (ORM) framework in C# that simplifies database access and management in web applications. By mapping database tables to C# objects and providing a high-level abstraction layer, Entity Framework Core streamlines data access operations. It reduces boilerplate code and promotes code consistency and maintainability. Entity Framework Core comes with features like migrations, change tracking and LINQ support. These features accelerate development workflows and enable developers to focus on building business logic rather than database interactions.
Dependency Injection
Dependency injection (DI) is a design pattern in C# that promotes loose coupling between components by dynamically injecting dependencies at runtime. By abstracting dependencies and decoupling components, DI enhances modularity, testability and extensibility in web applications. With frameworks like ASP.NET Core, developers can leverage built-in DI containers to manage object dependencies, facilitating inversion of control and promoting code maintainability, scalability and testability.
Significance of Advanced Concepts in C# Web Development
In the dynamic landscape of web development, mastering advanced concepts in C# is essential for developers aiming to stay ahead of the curve. Let’s dive deeper into why understanding these advanced concepts is essential:
Keeping Pace with Technological Advancements
The field of web development is constantly evolving, with new technologies, frameworks, and methodologies emerging at a rapid pace. By familiarizing themselves with advanced concepts in C#, developers can stay abreast of the latest trends and advancements in the industry. This allows them to leverage modern tools and techniques to build innovative web applications.
Enhancing Development Efficiency
Advanced concepts in C# often streamline the development process, enabling developers to write cleaner, more concise, and maintainable code. For example, features like LINQ (Language Integrated Query) allow developers to perform complex data queries with ease. This reduces the need for boilerplate code and enhancing productivity. Similarly, asynchronous programming techniques such as async/await facilitate non-blocking I/O operations, improving application responsiveness and performance.
Solving Complex Business Problems
Modern web applications often need to address complex business requirements and challenges. Advanced concepts in C# provide developers with powerful tools and frameworks to tackle these challenges effective. Whether it’s implementing robust security measures to protect sensitive data or optimizing application performance to handle large-scale traffic, developers benefit from a deep understanding of advanced C# concepts. Additionally, when integrating with external systems and services, this knowledge equips developers to architect elegant and scalable solutions.
Meeting User Expectations
In today’s digital age, users expect web applications to be fast, responsive and feature-rich. By harnessing the power of advanced concepts in C#, developers can build web applications that exceed user expectations. Whether it’s implementing real-time communication using SignalR, creating interactive user interfaces with Blazor or optimizing application performance with caching and load balancing techniques, advanced C# concepts enable developers to deliver superior user experiences.
Conclusion
In summary, gaining proficiency in advanced C# language features empowers developers to craft code that is more expressive, efficient and easily maintainable. By harnessing the capabilities of dependency injection, asynchronous programming, entity framework core, LINQ, developers enhance their abilities and produce robust, scalable and dependable applications in C#. Embracing these advanced features not only boosts developer productivity but also results in software solutions that are more resilient and performant.
At 8Seneca, we specialize in providing customized B2B services, with a focus on IT outsourcing solutions. Should you require assistance with IT outsourcing, please don’t hesitate to reach out to us. Furthermore, we’re currently welcoming enthusiastic interns to join our team. Visit our recruitment center for more information about available positions
Related Articles
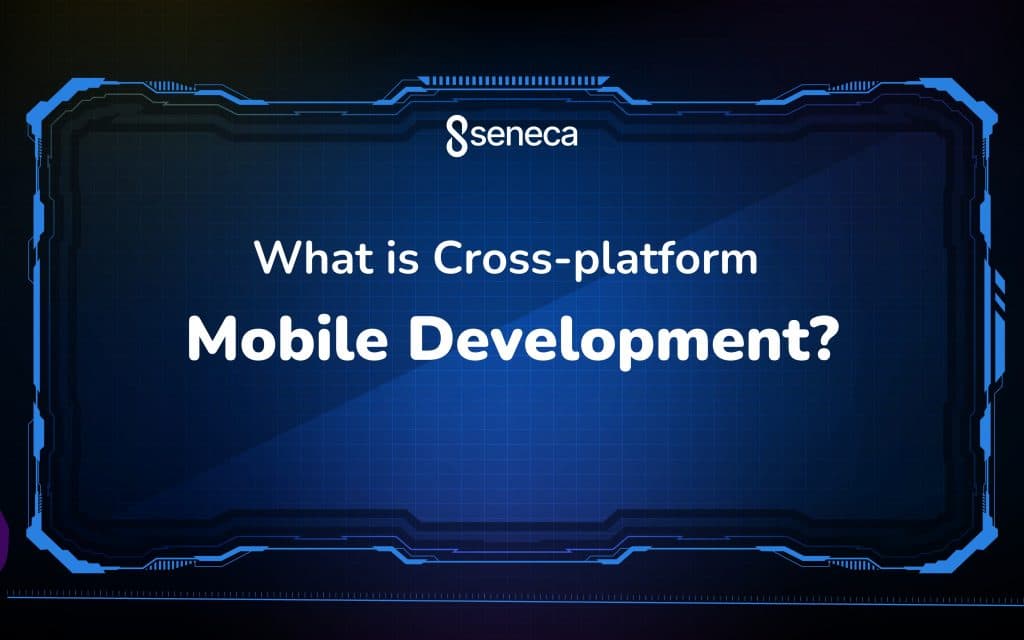
Dec 09, 2024
Read more
What is Cross-platform Mobile Development?
Learn what cross-platform mobile development is, its benefits, challenges, and popular frameworks like Flutter, React Native, and Xamarin.
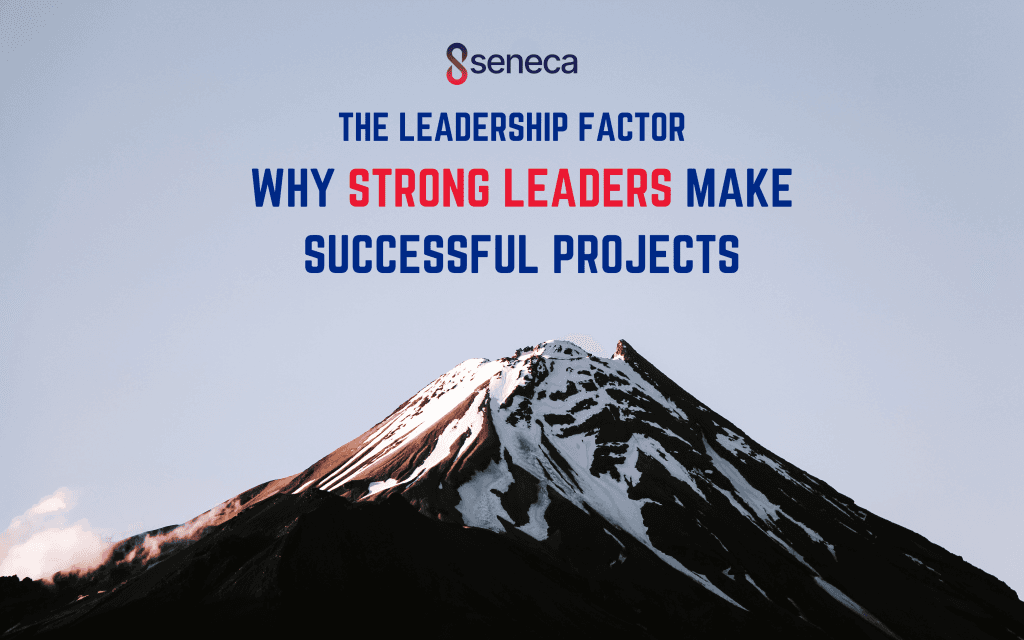
Dec 06, 2024
Read more
The Leadership Factor: Why Strong Leaders Make Successful Projects
Discover how effective leadership drives project success with strategies to inspire teams, tackle challenges, and achieve goals.
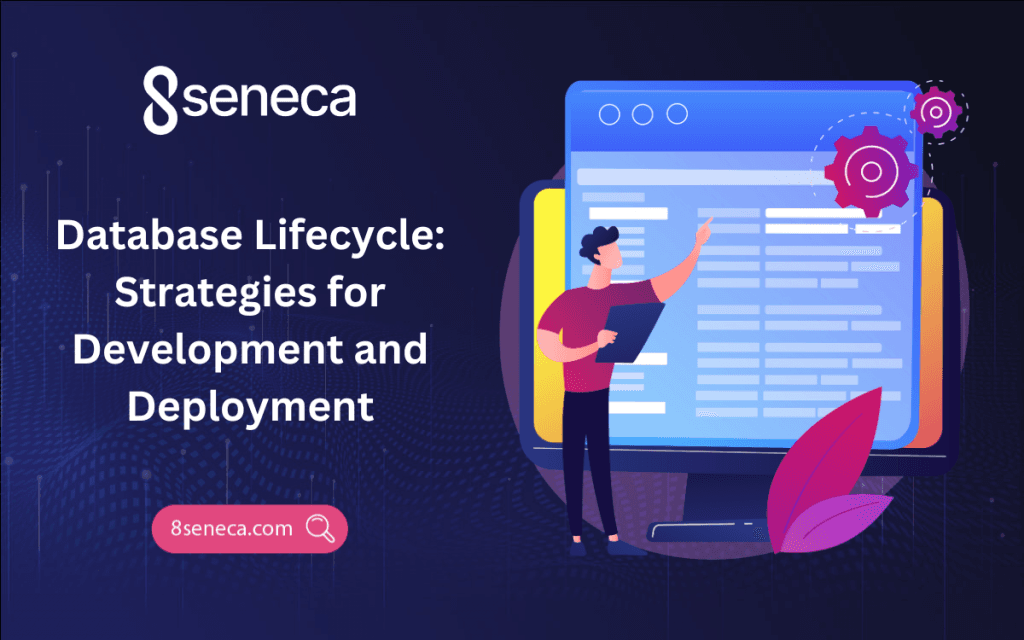
Oct 29, 2024
Read more
Database Lifecycle: Strategies for Development and Deployment
Learn the key stages of the database lifecycle—Planning, Analysis, Design, Implementation, Testing, Deployment, and Maintenance
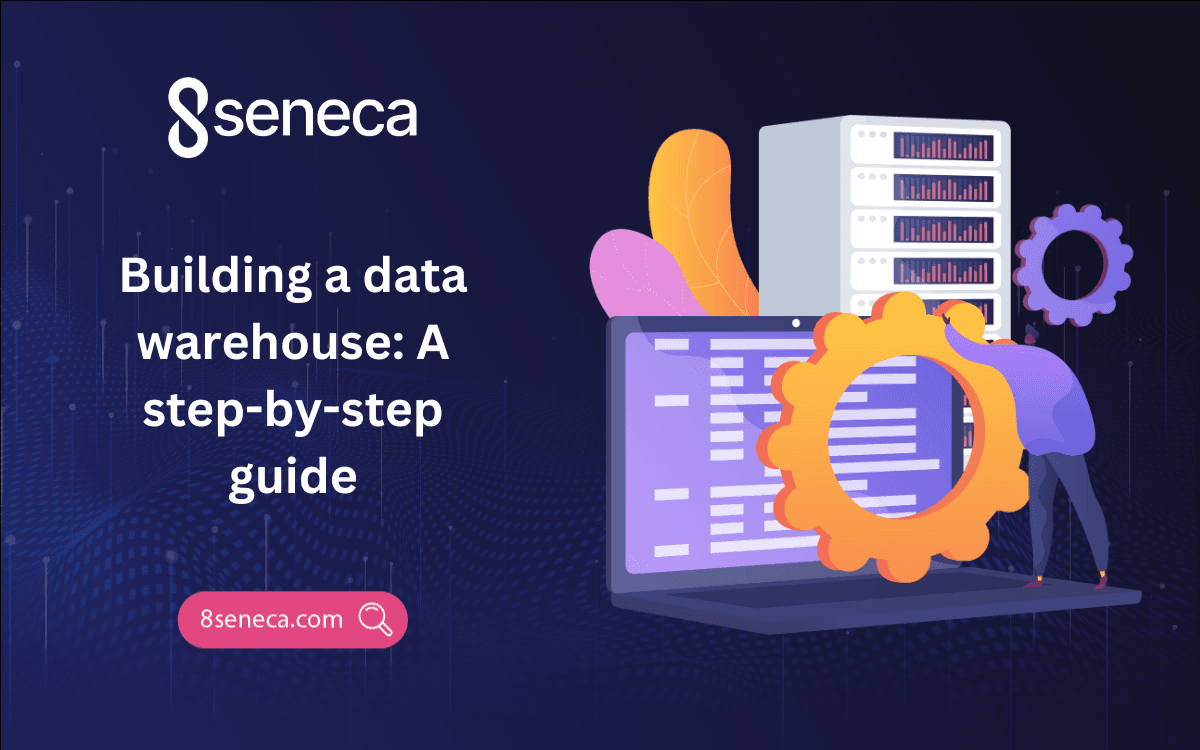
Sep 30, 2024
Read more
Building a Data Warehouse: A step-by-step guide
Learn the key components, best practices, challenges of building and maintaining a data warehouse, and how it can drive better business.
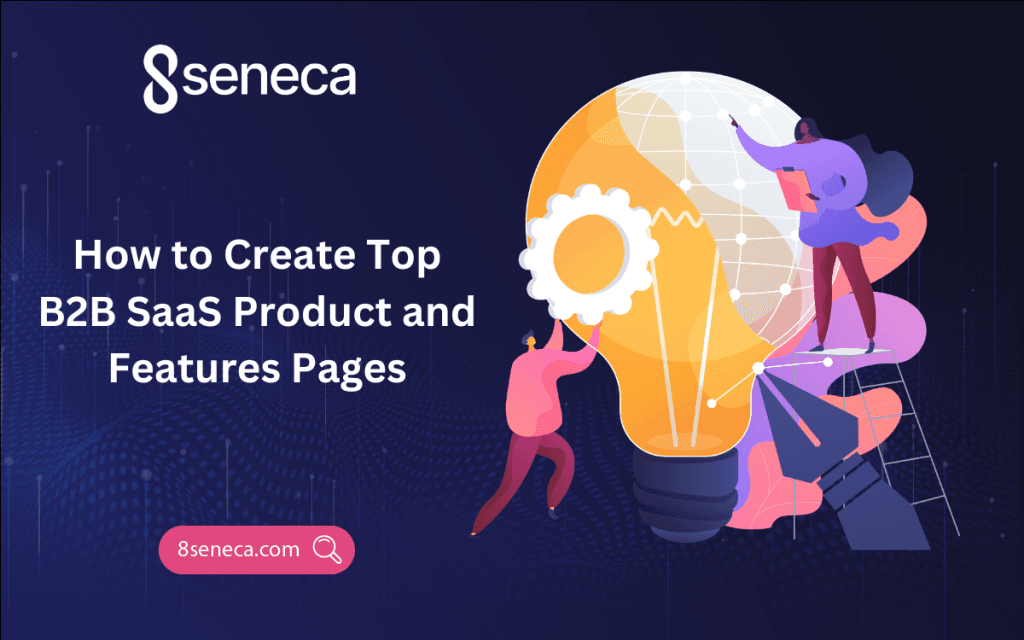
Sep 17, 2024
Read more
How to Create Top B2B SaaS Product and Features Pages
Explore key elements and examples of B2B SaaS product pages to boost conversions and showcase your product's value.
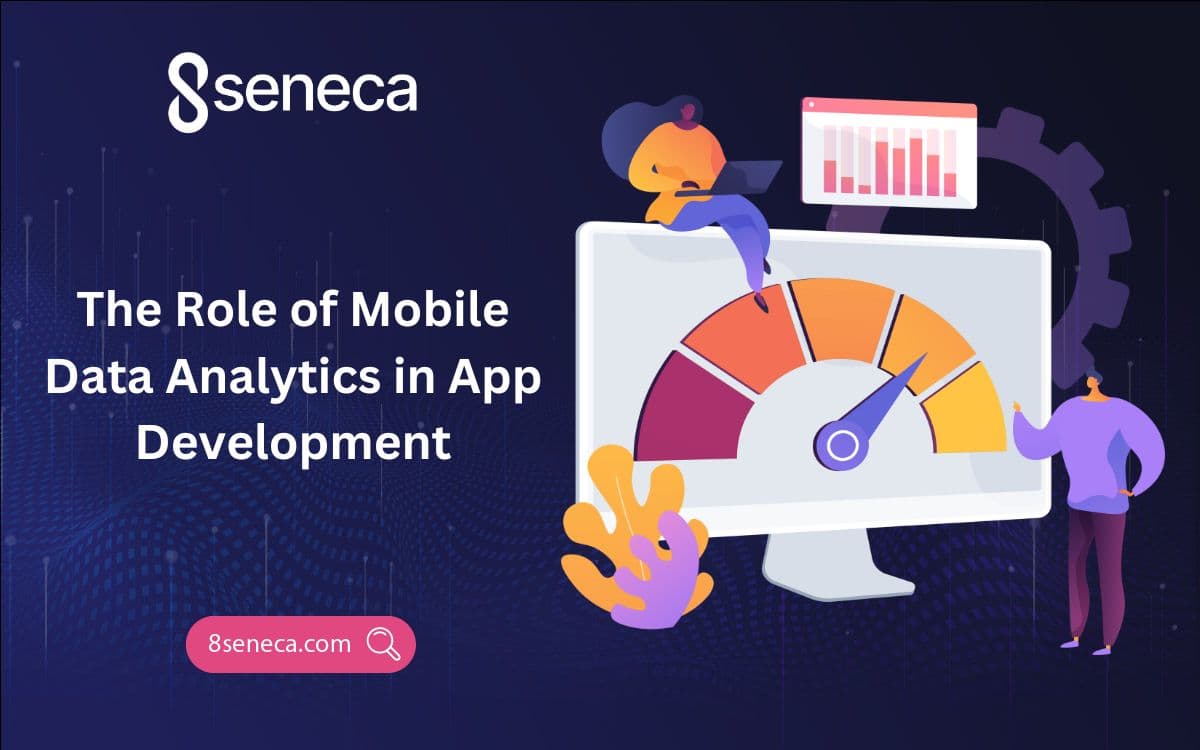
Sep 13, 2024
Read more
The Role of Mobile Data Analytics in App Development
Discover how mobile data analytics improves app development by enhancing user engagement, boosting retention, and optimizing performance.